Description
To find second largest element, first find the largest element. To find largest element, first declare a smallest element. max = Integer.MIN_VALUE Compare each element with this. If element larger than this is found, update max value. Continue this process until maximum element found. Now for finding second largest, again repeat the same process to find largest element but also check it should not be equal to maximum element(max).
C/C++
/* C program to print second largest element of the array*/
//Save it as SecondLargestElementArray.c
#include<stdio.h>
#include<limits.h>
int main(){
int i,n;
printf("Enter the size of array : ");
scanf("%d",&n);
//Declaring array
int arr[n];
printf("Enter the elements of the array : ");
for(i=0;i<n;i++) {
scanf("%d",&arr[i]);
}
//Declaring maximum element
int maximum = INT_MIN;
//Comparing with each element and find maximum element
for(i=0;i<n;i++) {
if(arr[i] > maximum) {
maximum = arr[i];
}
}
printf("The maximum value is : %d", maximum);
int second_max = INT_MIN;
//Finding Second largest element.
//Comparing with each element and also checking it is not equal to max
for(i=0;i<n;i++) {
if(arr[i] > second_max && arr[i]!=maximum) {
second_max = arr[i];
}
}
printf("\nThe second maximum value is : %d", second_max);
}
/* C program to print second largest element of the array*/
//Save it as SecondLargestElementArray.c
#include<stdio.h>
#include<limits.h>
int main(){
int i,n;
printf("Enter the size of array : ");
scanf("%d",&n);
//Declaring array
int arr[n];
printf("Enter the elements of the array : ");
for(i=0;i<n;i++) {
scanf("%d",&arr[i]);
}
//Declaring maximum element
int maximum = INT_MIN;
//Comparing with each element and find maximum element
for(i=0;i<n;i++) {
if(arr[i] > maximum) {
maximum = arr[i];
}
}
printf("The maximum value is : %d", maximum);
int second_max = INT_MIN;
//Finding Second largest element.
//Comparing with each element and also checking it is not equal to max
for(i=0;i<n;i++) {
if(arr[i] > second_max && arr[i]!=maximum) {
second_max = arr[i];
}
}
printf("\nThe second maximum value is : %d", second_max);
}
/* C program to print second largest element of the array*/ //Save it as SecondLargestElementArray.c #include<stdio.h> #include<limits.h> int main(){ int i,n; printf("Enter the size of array : "); scanf("%d",&n); //Declaring array int arr[n]; printf("Enter the elements of the array : "); for(i=0;i<n;i++) { scanf("%d",&arr[i]); } //Declaring maximum element int maximum = INT_MIN; //Comparing with each element and find maximum element for(i=0;i<n;i++) { if(arr[i] > maximum) { maximum = arr[i]; } } printf("The maximum value is : %d", maximum); int second_max = INT_MIN; //Finding Second largest element. //Comparing with each element and also checking it is not equal to max for(i=0;i<n;i++) { if(arr[i] > second_max && arr[i]!=maximum) { second_max = arr[i]; } } printf("\nThe second maximum value is : %d", second_max); }
Output
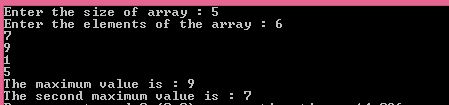
Java
/*Java program to print second largest element of the array*/
//Save it as SecondLargestElementArray.java
import java.io.*;
import java.util.Scanner;
public class SecondLargestElementArray {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the size of array : ");
int n = scanner.nextInt();
//Declaring array
int arr[] = new int[n];
System.out.println("Enter the elements of the array : ");
for(int i=0;i<n;i++) {
arr[i] = scanner.nextInt();
}
//Declaring maximum element
int max = Integer.MIN_VALUE;
//Comparing with each element and find maximum element
for(int i=0;i<n;i++) {
if(arr[i] > max) {
max = arr[i];
}
}
System.out.println("The maximum value is : " + max);
int second_max = Integer.MIN_VALUE;
//Finding Second largest element.
//Comparing with each element and also checking it is not equal to max
for(int i=0;i<n;i++) {
if(arr[i] > second_max && arr[i]!=max) {
second_max = arr[i];
}
}
System.out.println("The second maximum value is : " + second_max);
}
}
/*Java program to print second largest element of the array*/
//Save it as SecondLargestElementArray.java
import java.io.*;
import java.util.Scanner;
public class SecondLargestElementArray {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the size of array : ");
int n = scanner.nextInt();
//Declaring array
int arr[] = new int[n];
System.out.println("Enter the elements of the array : ");
for(int i=0;i<n;i++) {
arr[i] = scanner.nextInt();
}
//Declaring maximum element
int max = Integer.MIN_VALUE;
//Comparing with each element and find maximum element
for(int i=0;i<n;i++) {
if(arr[i] > max) {
max = arr[i];
}
}
System.out.println("The maximum value is : " + max);
int second_max = Integer.MIN_VALUE;
//Finding Second largest element.
//Comparing with each element and also checking it is not equal to max
for(int i=0;i<n;i++) {
if(arr[i] > second_max && arr[i]!=max) {
second_max = arr[i];
}
}
System.out.println("The second maximum value is : " + second_max);
}
}
/*Java program to print second largest element of the array*/ //Save it as SecondLargestElementArray.java import java.io.*; import java.util.Scanner; public class SecondLargestElementArray { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter the size of array : "); int n = scanner.nextInt(); //Declaring array int arr[] = new int[n]; System.out.println("Enter the elements of the array : "); for(int i=0;i<n;i++) { arr[i] = scanner.nextInt(); } //Declaring maximum element int max = Integer.MIN_VALUE; //Comparing with each element and find maximum element for(int i=0;i<n;i++) { if(arr[i] > max) { max = arr[i]; } } System.out.println("The maximum value is : " + max); int second_max = Integer.MIN_VALUE; //Finding Second largest element. //Comparing with each element and also checking it is not equal to max for(int i=0;i<n;i++) { if(arr[i] > second_max && arr[i]!=max) { second_max = arr[i]; } } System.out.println("The second maximum value is : " + second_max); } }
Output

Other Methods(By Sorting)
To find second largest element, sort the array either ascending or descending order. 1) If array is sorted in ascending order then second element from end will be the second largest element of array. 2) If array is sorted in descending order then second element from beginning will be the second largest element of array. For sorting, follow any of the sorting algorithm based on requirement. For sorting, array function Arrays.sort(arrayname) can also be used. It will sort array in ascending order.
C/C++
//save as second_largest_element_sorting.c
#include<stdio.h>
int main(){
int i,n;
printf("Enter the size of array : ");
scanf("%d",&n);
int arr[n];
printf("Enter elements : ");
for(i=0;i<n;i++){
scanf("%d",&arr[i]);
}
printf("The array elements are : ");
for(i=0;i<n;i++){
printf("%d ",arr[i]);
}
//Calling function to sort the array
array_sort(arr,n);
printf("\nThe array after sorting : ");
for(i=0;i<n;i++){
printf("%d ",arr[i]);
}
/*As array is sorted in ascending order then second element from end
will be the second largest element of array.*/
printf("\nSecond largest element is : %d",arr[n-2]);
return 0;
}
//Using bubble sort algorithm for sorting
void array_sort(int arr[],int n){
int i,j,temp;
for(i=0;i<n-1;i++){
for(j=(i+1);j<n;j++) {
if(arr[i] > arr[j]) {
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
//save as second_largest_element_sorting.c
#include<stdio.h>
int main(){
int i,n;
printf("Enter the size of array : ");
scanf("%d",&n);
int arr[n];
printf("Enter elements : ");
for(i=0;i<n;i++){
scanf("%d",&arr[i]);
}
printf("The array elements are : ");
for(i=0;i<n;i++){
printf("%d ",arr[i]);
}
//Calling function to sort the array
array_sort(arr,n);
printf("\nThe array after sorting : ");
for(i=0;i<n;i++){
printf("%d ",arr[i]);
}
/*As array is sorted in ascending order then second element from end
will be the second largest element of array.*/
printf("\nSecond largest element is : %d",arr[n-2]);
return 0;
}
//Using bubble sort algorithm for sorting
void array_sort(int arr[],int n){
int i,j,temp;
for(i=0;i<n-1;i++){
for(j=(i+1);j<n;j++) {
if(arr[i] > arr[j]) {
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
//save as second_largest_element_sorting.c #include<stdio.h> int main(){ int i,n; printf("Enter the size of array : "); scanf("%d",&n); int arr[n]; printf("Enter elements : "); for(i=0;i<n;i++){ scanf("%d",&arr[i]); } printf("The array elements are : "); for(i=0;i<n;i++){ printf("%d ",arr[i]); } //Calling function to sort the array array_sort(arr,n); printf("\nThe array after sorting : "); for(i=0;i<n;i++){ printf("%d ",arr[i]); } /*As array is sorted in ascending order then second element from end will be the second largest element of array.*/ printf("\nSecond largest element is : %d",arr[n-2]); return 0; } //Using bubble sort algorithm for sorting void array_sort(int arr[],int n){ int i,j,temp; for(i=0;i<n-1;i++){ for(j=(i+1);j<n;j++) { if(arr[i] > arr[j]) { temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } } }
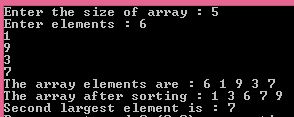
Java
//Save as SecondLargestElementSorting.java
import java.io.*;
import java.util.Scanner;
public class SecondLargestElementSorting {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int i, n;
System.out.println("Enter the size of array : ");
n = scanner.nextInt();
int arr[] = new int[n];
System.out.println("Enter elements : ");
for (i = 0; i < n; i++) {
arr[i] = scanner.nextInt();
}
System.out.println("The elements of array are : ");
for (i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
//Calling function to sort the array
bubbleSort(arr, n);
System.out.println("\nThe Sorted array is : ");
for (i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
/*As array is sorted in ascending order then second element from end
will be the second largest element of array.*/
System.out.println("\nSecond largest element is : "+arr[n-2]);
}
//Using bubble sort algorithm for sorting
private static void bubbleSort(int[] arr, int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = (i + 1); j < n; j++) {
if (arr[i] > arr[j]) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
}
//Save as SecondLargestElementSorting.java
import java.io.*;
import java.util.Scanner;
public class SecondLargestElementSorting {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int i, n;
System.out.println("Enter the size of array : ");
n = scanner.nextInt();
int arr[] = new int[n];
System.out.println("Enter elements : ");
for (i = 0; i < n; i++) {
arr[i] = scanner.nextInt();
}
System.out.println("The elements of array are : ");
for (i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
//Calling function to sort the array
bubbleSort(arr, n);
System.out.println("\nThe Sorted array is : ");
for (i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
/*As array is sorted in ascending order then second element from end
will be the second largest element of array.*/
System.out.println("\nSecond largest element is : "+arr[n-2]);
}
//Using bubble sort algorithm for sorting
private static void bubbleSort(int[] arr, int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = (i + 1); j < n; j++) {
if (arr[i] > arr[j]) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
}
//Save as SecondLargestElementSorting.java import java.io.*; import java.util.Scanner; public class SecondLargestElementSorting { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int i, n; System.out.println("Enter the size of array : "); n = scanner.nextInt(); int arr[] = new int[n]; System.out.println("Enter elements : "); for (i = 0; i < n; i++) { arr[i] = scanner.nextInt(); } System.out.println("The elements of array are : "); for (i = 0; i < n; i++) { System.out.print(arr[i] + " "); } //Calling function to sort the array bubbleSort(arr, n); System.out.println("\nThe Sorted array is : "); for (i = 0; i < n; i++) { System.out.print(arr[i] + " "); } /*As array is sorted in ascending order then second element from end will be the second largest element of array.*/ System.out.println("\nSecond largest element is : "+arr[n-2]); } //Using bubble sort algorithm for sorting private static void bubbleSort(int[] arr, int n) { int i, j; for (i = 0; i < n - 1; i++) { for (j = (i + 1); j < n; j++) { if (arr[i] > arr[j]) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } } } }
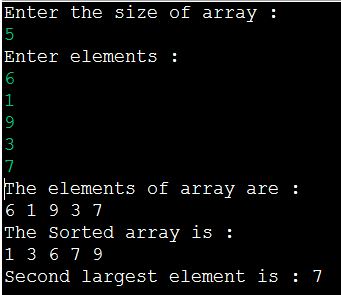
Related Programs
1) Find Smallest element and it’s position of an Array2) Program to insert a number at given position of an Array
3) Program to delete an element from given location in an array
4)Program to delete an element from an array sorted in ascending order
5) Program to swap maximum and minimum element of Array
6) Program to Search for an Element in an Array
7) Program to Reverse an Array using Recursion
8) Program to Find Median of a Array
9) Program to Find the smallest missing number
10) Program to Find K largest elements from array
11) Program to Check Array is Perfect or Not
12) Remove Duplicate Elements From Array