Description
Linked List representation of stack is difficult to implement but there is no need to declare the size before. Addition and deletion of node from stack can be done on node pointed by TOP. Here all three operation like push, pop and peek can be performed. 1)PUSH : PUSH operation is used to insert node into the stack. case 1 : If stack do not have any node Make the new node as first node and top will point to this new node. case 2 : If stack has node Make the new node as first node of stack by adding address of top to the next field of new node and assigning the address of new node to top. 2) POP : POP operation is used to delete node from stack case 1 : If stack do not have node Show message stack underflow case 2 : If stack has node The node pointed by top will be deleted and top will point to node next to node pointed by top. 3) PEEK : PEEK operation does not insert or delete any node to stack. case 1 : If stack do not have node Show message stack underflow case 2 : If stack has node Show the node pointed by top.
C/C++
#include<stdio.h> #include<malloc.h> void *push(); void *pop(); void *peek(); void *display(); //Declaring nodes for stack struct stack{ int data; struct stack *next; }; struct stack *top = NULL; int main(){ int option; do{ printf("\nWhich operation you want to perform : "); printf("\n1.PUSH\n2.POP\n3.PEEK\n4.DISPLAY\n5.EXIT : \n"); scanf("%d",&option); switch(option){ case 1: push(); break; case 2: pop(); break; case 3: peek(); break; case 4: display(); break; case 5: break; default: printf("Enter Valid Input"); } }while(option!=5); return 0; } void *push(){ int val; printf("Enter the value to be added to stack : "); scanf("%d",&val); //Creating new node struct stack *newNode = (struct stack *)malloc(sizeof(struct stack)); newNode->data = val; //If stack has no any element if(top==NULL){ newNode->next=NULL; top=newNode; }else{ //If stack has few elements newNode->next=top; top=newNode; } } void *pop(){ struct stack *temp; //If stack is empty if(top==NULL){ printf("Stack Underflow"); }else{ //If stack has elements temp=top; top=top->next; printf("The poped value is : %d",temp->data); free(temp); } } //In peek operation stack return the top element without deleting any element void *peek(){ //if stack is empty if(top==NULL){ printf("Stack Underflow"); }else{ //If stack has elements printf("The peek value is : %d",top->data); } } //It display the elements of stack void *display(){ //If stack is empty if(top==NULL){ printf("Stack Underflow"); }else{ //If stack has elements then show from top struct stack *temp = top; printf("The elements of stack : "); while(temp!=NULL){ printf("%d ",temp->data); temp=temp->next; } } }
Output
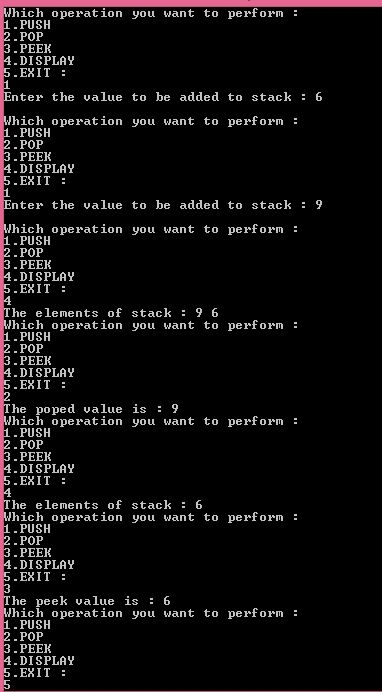
Java
import java.io.*; import java.util.Scanner; public class LinkedListImplStack { //Declaring nodes for stack static class Stack{ int data; Stack next; public Stack(int data) { this.data = data; this.next = null; } } public static Stack top = null; static Scanner scanner = new Scanner(System.in); public static void main(String[] args) { int option; do { System.out.println("\nWhich operation you want to perform : "); System.out.println("1.PUSH\n2.POP\n3.PEEK\n4.DISPLAY\n5.EXIT : "); option = scanner.nextInt(); switch (option) { case 1: push(); break; case 2: pop(); break; case 3: peek(); break; case 4: display(); break; case 5: System.out.println("Program terminated"); break; default: System.out.println("Enter Valid Input"); } } while(option!=5); } //It display the elements of stack private static void display() { //If stack is empty if (top == null) { System.out.println("**Stack is empty**"); } else { //If stack has elements then show from top Stack temp = top; System.out.println("The elements of stack : "); while(temp!=null){ System.out.print(temp.data+" "); temp=temp.next; } } } //In peek operation stack return the top element without deleting any element private static void peek() { //if stack is empty if(top==null){ System.out.println("Stack Underflow"); }else{ //If stack has elements System.out.println("The peek value is : "+top.data); } } private static void pop() { Stack temp; //If stack is empty if(top==null){ System.out.println("Stack Underflow"); }else{ //If stack has elements temp=top; top=top.next; System.out.println("The poped value is : "+temp.data); } } private static void push() { int val; System.out.println("Enter the value to be added to stack : "); val = scanner.nextInt(); //Creating new node Stack newNode = new Stack(val); //If stack has no any element if(top==null){ newNode.next=null; top=newNode; }else{ //If stack has few elements newNode.next=top; top=newNode; } } }
Output
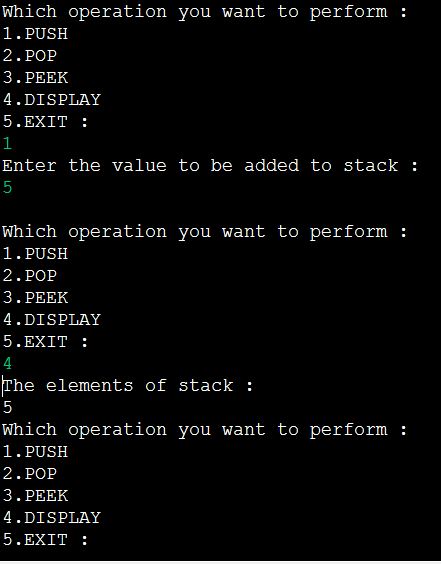
Related Programs
1. What is Stack2. Array Representation Of Stack
3. Program To Create And Display Singly Linked List