Description
To reverse the elements of stack first insert all elements of array into stack. Now pop the elements of stack one by one and store into array. As stack is LIFO(Last In First Out) so after pop when the elements of stack will be stored in array then last element of previous array will be stored first into new array. Print the element of new array that will be the reversed array.
C/C++
//save as reverse_array_stack.c #include<stdio.h> int stack[100]; int TOP=-1; void push(int); int pop(); int main(){ int i,n,data; printf("Enter the size of array : "); scanf("%d",&n); int arr[n]; printf("Enter the elements of array : "); for(i=0;i<n;i++){ scanf("%d",&arr[i]); //Inserting elements of array into stack push(arr[i]); } printf("The elements of array : "); for(i=0;i<n;i++){ printf("%d ",arr[i]); } //storing the popped elements of stack into array for(i=0;i<n;i++){ data = pop(); arr[i] = data; } //Printing the elements of array after reversal printf("\nThe reverse of array : "); for(i=0;i<n;i++){ printf("%d ",arr[i]); } return 0; } //Function to insert elements of array into stack void push(int data){ TOP++; stack[TOP]=data; } //Function to pop the element of stack int pop(){ int val = stack[TOP]; TOP--; return val; }
Output
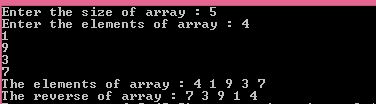
Java
//Save as ReverseArrayUsingStack.java import java.util.Scanner; public class ReverseArrayUsingStack { static int TOP = -1; static int stack[] = new int[100]; public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter the size of array : "); int n = scanner.nextInt(); int arr[] = new int[n]; System.out.println("Enter the elements of the array : "); for (int i = 0; i < n; i++) { arr[i] = scanner.nextInt(); //Inserting elements of array into stack push(arr[i]); } System.out.println("The elements of array : "); for(int i=0;i<n;i++) { System.out.print(arr[i]+" "); } //storing the popped elements of stack into array for(int i=0;i<n;i++) { int data = pop(); arr[i]=data; } //Printing the elements of array after reversal System.out.println("\nThe reverse of array : "); for(int i=0;i<n;i++) { System.out.print(arr[i]+" "); } } //Function to insert elements of array into stack private static int pop() { int data = stack[TOP]; TOP--; return data; } //Function to pop the element of stack private static void push(int data) { TOP++; stack[TOP] = data; } }
Output
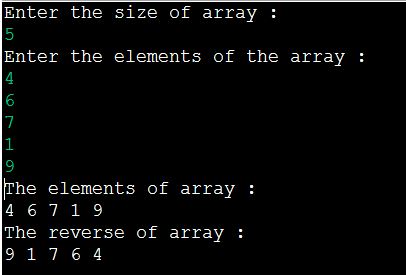
Related Programs
1. What is Stack2. Array Representation Of Stack
3. Linked List Representation Of Stack