Description
The array implementation of stack means all the operations of stack will be performed using array. Here all the operation means push, pop, peek. 1) PUSH : Push operation means insertion of element into stack. Before push operation, first check if stack is full or not. If full show overflow message. otherwise, increase the index by one and insert the element to that index. 2) POP : Pop operation means deletion of a element from stack. Before pop operation, first check stack is empty or not. If empty show underflow message. otherwise, the element present at top will be pop element and decrease the index of stack by one. 3) PEEK : In peek operation, there will not any operation performed it just return top element of stack. If there is not any element then show underflow message. Advantage of array implementation stack is it is easy to implement and disadvantage is the size of array cannot increment after declaration.
C/C++
/*c program to represent array as stack*/ //Save as array_stack.c #include<stdio.h> int stack[100],n,option,TOP=-1; void push(); void pop(); void peek(); void display(); int main(){ printf("Enter the size of stack : "); scanf("%d",&n); do{ printf("\nWhich operation you want to perform : "); printf("\n1.PUSH\n2.POP\n3.PEEK\n4.DISPLAY\n5.EXIT : \n"); scanf("%d",&option); switch(option){ case 1: push(); break; case 2: pop(); break; case 3: peek(); break; case 4: display(); break; case 5: break; default: printf("Enter Valid Input"); } }while(option!=5); return 0; } void push(){ //If stack is full show overflow message if(TOP==n-1){ printf("**Overflow**"); }else{ //If stack has free space then increment the index by one and insert the element int val; printf("Enter the value to be added to stack : "); scanf("%d",&val); TOP=TOP+1; stack[TOP]=val; } } void pop(){ //If stack is empty show underflow message if(TOP==-1){ printf("**Underflow**"); }else{ //If stack has element then topmost element will be popped printf("The poped value is : %d",stack[TOP]); //Decrement the index by one TOP=TOP-1; } } //In peek operation, there will not any operation performed it just return top element of stack. void peek(){ //If stack is empty show underflow message if(TOP==-1){ printf("**Stack is empty**"); }else{ //If stack has element then topmost element will be peek element printf("The value present on top of the stack : %d",stack[TOP]); } } //Function for displaying elements of stack void display(){ //If stack is empty show underflow message if(TOP==-1){ printf("**Stack is empty**"); }else{ int i; printf("The elements of stack is : "); //Displaying elements from last to first for(i=TOP;i>=0;i--){ printf("%d ",stack[i]); } } }
Output
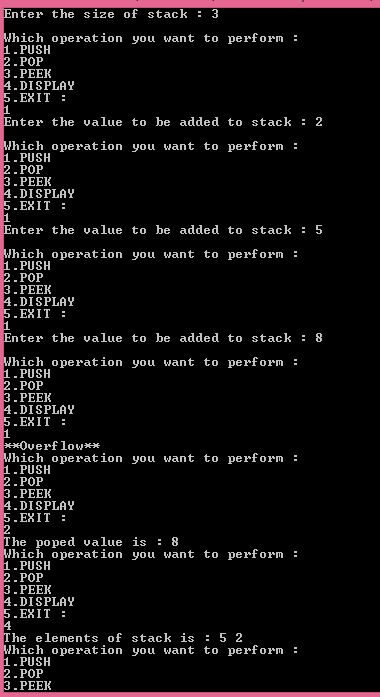
Java
/*java program to represent array as stack*/ //Save as ArrayImplStack.java import java.io.*; import java.util.Scanner; public class ArrayImplStack { static int n; static int TOP = -1; static int stack[] = new int[100]; static Scanner scanner = new Scanner(System.in); public static void main(String[] args) { int option; System.out.println("Enter the size of stack : "); n = scanner.nextInt(); do { System.out.println("\nWhich operation you want to perform : "); System.out.println("1.PUSH\n2.POP\n3.PEEK\n4.DISPLAY\n5.EXIT : "); option = scanner.nextInt(); switch (option) { case 1: push(); break; case 2: pop(); break; case 3: peek(); break; case 4: display(); break; case 5: break; default: System.out.println("Enter Valid Input"); } } while(option!=5); } //Method for displaying elements of stack private static void display() { //If stack is empty show underflow message if (TOP == -1) { System.out.println("**Stack is empty**"); } else { int i; System.out.println("The elements of stack is : "); //Displaying elements from last to first for (i = TOP; i >= 0; i--) { System.out.print(stack[i] + " "); } } } //In peek operation, there will not any operation performed it just return top element of stack. private static void peek() { //If stack is empty show underflow message if (TOP == -1) { System.out.println("**Stack is empty**"); } else { //If stack has element then topmost element will be peek element System.out.println("The value present on top of the stack : " + stack[TOP]); } } private static void pop() { //If stack is empty show underflow message if (TOP == -1) { System.out.println("**Underflow**"); } else { //If stack has element then topmost element will be popped System.out.println("The poped value is : " + stack[TOP]); //Decrement the index by one TOP = TOP - 1; } } private static void push() { //If stack is full show overflow message if (TOP == n - 1) { System.out.println("**Overflow**"); } else { //If stack has free space then increment the index by one and insert the element int val; System.out.println("Enter the value to be added to stack : "); val = scanner.nextInt(); TOP = TOP + 1; stack[TOP] = val; } } }
Output
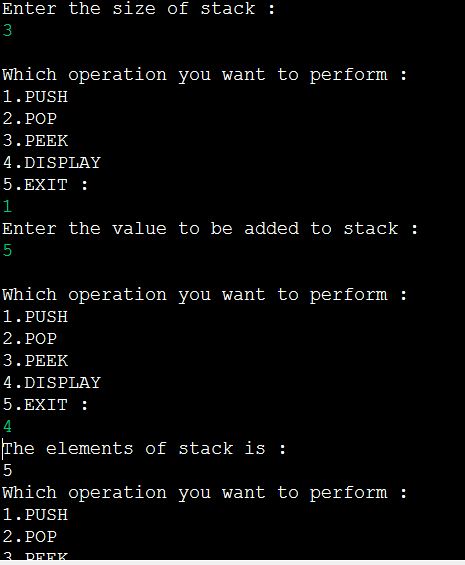
Related Programs
1. What is Stack2. Array Example
3. String Example
4. Linked List Example